HIVE provides a function that can be used as a game alarm by registering a push notification to a device from a game client without HIVE push server. This is called Local Push. HIVE provides local push registration and release functions. The registered local push will notify you at the specified time even if you close your app, and the registered local push can be unregistered as needed.
Local Push Data
HIVE defines Local Push data in LocalPush class with the following contents.
Name | Type | Description |
---|---|---|
noticeId | Integer | Identifier of Local Push message |
title | String | Title of Local Push message |
msg | String | Contents of Local Push message |
after | Integer | Interval between push registration and message popup (unit: second, default=0) |
groupId | String | This is the group ID for notification group. The notification group displays the notifications sent from the same app in a group on a user’s device. If you didn’t set a value for this, the local push messages would be displayed in the default app group. |
Registering Local Push
To register Local Push Notification on user devices, call registerLocalPush()
method of Push class.
On iOS, the maximum count of Local Push Notification for registration is 64, and it may limit up to less than 64 according to the version of the device and OS. If you register notifications over the limitation, it discards the oldest notification in order. (Link)
Followings are sample codes to register Local Push.
API Reference: hive.Push.registerLocalPush
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
using hive; LocalPush localPush = new LocalPush (); localPush.noticeId = 1; localPush.title = "Local Push Title"; localPush.msg = "Local Push Message"; localPush.after = 5; localPush.groupId = "a"; Push.registerLocalPush(localPush, (ResultAPI result, LocalPush localPush) => { if (result.isSuccess()) { // call successful } }); |
API Reference: Push::registerLocalPush
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
#include <HIVE_SDK_Plugin/HIVE_CPP.h> using namespace std; using namespace hive; LocalPush localPush; localPush.noticeId = 1; localPush.title = "Local Push Title"; localPush.msg = "Local Push Message"; localPush.after = 5; localPush.groupId = "a"; Push::registerLocalPush(localPush, [=](ResultAPI result, LocalPush localPush){ if (result.isSuccess()) { // call successful } }); |
API Reference: registerLocalPush
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
import com.hive.Push import com.hive.ResultAPI val localPush = Push.LocalPush().apply { noticeID = 1 title = "Local Push Title" msg = "Local Push Message" after = 5 groupId = "a" } Push.registerLocalPush(localPush, object : Push.LocalPushListener { override fun onRegisterLocalPush(result: ResultAPI, localPush: Push.LocalPush?) { if (result.isSuccess) { // call successful } } }) |
API Reference: com.hive.Push.registerLocalPush
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
import com.hive.Push; import com.hive.ResultAPI; Push.LocalPush localPush = new Push.LocalPush(); localPush.setNoticeID(1); localPush.setTitle("Local Push Title"); localPush.setMsg("Local Push Message"); localPush.setAfter(5); localPush.setGroupId("a"); Push.INSTANCE.registerLocalPush(localPush, (result, localPushData) -> { if (result.isSuccess()) { // call successful } }); |
API Reference: registerLocalPush(_:handler:)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
import HIVEService let localPush = LocalPush() localPush.noticeId = 1; localPush.title = "Local Push Title"; localPush.msg = "Local Push Message"; localPush.after = 5; localPush.groupId = "a"; PushInterface.registerLocalPush(localPush) { result, localPush in if result.isSuccess() { // call successful } } |
API Reference: Objective-C
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
#import <HIVEService/HIVEService-Swift.h> HIVELocalPush *localPush = [[HIVELocalPush alloc] init]; localPush.noticeId = 1; localPush.title = @"Local Push Title"; localPush.msg = @"Local Push Message"; localPush.after = 5; localPush.groupId = @"a"; [HIVEPush registerLocalPush: localPush handler: ^(HIVEResultAPI *result, HIVELocalPush *localPush) { if ([result isSuccess]) { // call successful } }]; |
Unregistering Local Push
You can unregister the registered local push notification before the specified notification time.
Unregister the Registered Local Push
Using the following APIs, set the registered push identifier as parameter (noticeId), and unregister the local push.
API Reference: hive .Push.unregisterLocalPush
1 2 3 4 5 |
using hive; int noticeId = 1; // local push unique ID Push.unregisterLocalPush(noticeId); |
API Reference: Push ::unregisterLocalPush
1 2 3 4 5 6 |
#include <HIVE_SDK_Plugin/HIVE_CPP.h> using namespace std; using namespace hive; int noticeId = 1; // local push unique ID Push::unregisterLocalPush(noticeId); |
API Reference: Push.unregisterLocalPush
1 2 3 4 |
import com.hive.Push val noticeId = 1 // local push unique ID Push.unregisterLocalPush(noticeId) |
API Reference: com .hive.Push.unregisterLocalPush
1 2 3 4 |
import com.hive.Push; int noticeId = 1; // local push unique ID Push.INSTANCE.unregisterLocalPush(noticeId); |
API Reference: PushInterface .unregisterLocalPush
1 2 3 4 |
import HIVEService let noticeId = 1 // local push unique ID PushInterface.unregisterLocalPush(noticeId) |
API Reference: HIVEPush ::unregisterLocalPush
1 2 3 4 |
#import <HIVEService/HIVEService-Swift.h> NSInteger noticeId = 0; // local push unique ID [HIVEPush unregisterLocalPush: noticeId]; |
Unregister the Listed Local Pushes at once
Using the following APIs, you can create the list of registered local push identifier, and unregister the local push at once.
API Reference: hive.Push .unregisterLocalPushes
1 2 3 4 5 |
using hive; List noticeIds = new List { 1, 2, 3 }(); Push.unregisterLocalPushes(noticeIds); |
API Reference: Push:: unregisterLocalPushes
1 2 3 4 5 6 7 |
#include <HIVE_SDK_Plugin/HIVE_CPP.h> using namespace std; using namespace hive; vector noticeIds = { 1, 2, 3 }; Push::unregisterLocalPushes(noticeIds); |
API Reference: Push.unregisterLocalPushes
1 2 3 4 5 |
import com.hive.Push val noticeIds = arrayListOf(1, 2, 3) Push.unregisterLocalPushes(noticeIds) |
API Reference: com.hive .Push.unregisterLocalPushes
1 2 3 4 5 6 7 8 |
import com.hive.Push; ArrayList<Integer> noticeIds = new ArrayList<>(); noticeIds.add(1); noticeIds.add(2); noticeIds.add(3); Push.INSTANCE.unregisterLocalPushes(noticeIds); |
API Reference: PushInterface .unregisterLocalPushes
1 2 3 4 5 |
import HIVEService let noticeIds = [1, 2, 3] PushInterface.unregisterLocalPushes(noticeids) |
API Reference: HIVEPush:: unregisterLocalPushes
1 2 3 4 5 |
#import <HIVEService/HIVEService-Swift.h> NSArray* noticeIds = @[ @(1), @(2), @(3) ]; [HIVEPush unregisterLocalPushes: noticeIds]; |
Unregister all Local Pushes
Using the following APIs, you can unregister not only Hive’s local pushes but also all local pushes in listening state.
API Reference: hive.Push .unregisterAllLocalPushes
1 2 3 |
using hive; Push.unregisterAllLocalPushes(); |
API Reference: Push:: unregisterAllLocalPushes
1 2 3 4 5 |
#include <HIVE_SDK_Plugin/HIVE_CPP.h> using namespace std; using namespace hive; Push::unregisterAllLocalPushes(); |
API Reference: Push.unregisterAllLocalPushes
1 2 3 |
import com.hive.Push Push.unregisterAllLocalPushes() |
API Reference: com.hive .Push.unregisterAllLocalPushes
1 2 3 |
import com.hive.Push; Push.INSTANCE.unregisterAllLocalPushes(); |
API Reference: PushInterface.unregisterAllLocalPushes
1 2 3 |
import HIVEService PushInterface.unregisterAllLocalPushes() |
API Reference: HIVEPush:: unregisterAllLocalPushes
1 2 3 |
#import <HIVEService/HIVEService-Swift.h> [HIVEPush unregisterAllLocalPushes]; |
Customizing Local Push UI (Android only)
Local Push can be customized on Android device. Information about the UI elements to be customized is set in the LocalPush class parameter of the registerLocalPush()
method that registers the local push along with the push setting information.
Local Push UI
The customizing data of Local Push UI is defined in the LocalPush class, which defines the data of Local Push Settings. Following table describes some changeable fields from LocalPush class relevant to UI items.
Some field names are linked with details. Click the field name to read more details with screen shot.
Name | Type | Description | Required |
---|---|---|---|
type |
String | The dialog type of the push message:
|
Optional |
bigmsg | String | Contents which users peek from the top of the screen. Unlimited length Caution: Available with Android Jelly Bean (4.1) and higher |
Optional |
ticker | String | Tickers with push notifications | Optional |
icon | String | The file name in /res/drawable directory , excluding file extension. e.g. If the file path is res/drawable-xhdpi/hive_icon.png, the value of icon field is "hive_icon" .Without file name, game icon is displayed as default |
Optional |
sound | String | File path for sound file alarming push notifications Without file path, default notification sound |
Optional |
active | String | Action settings when users tap a push notification:
|
Optional |
broadcastAction | String | Action value to broadcast | Optional |
buckettype | Integer | Type to display push notifications:
|
Optional |
bucketsize | Integer | The number of messages related to one push ID to show at once | Optional |
bigpicture | String | File path for image file displaying on push notifications | Optional /td> |
icon_color | String | Background color of icon Format: {"r":[0–255],"g":[0–255],"b":[0–255]} e.g.: {"r":0,"g":128,"b":255} Caution: Available with Android Lollipop (5.0) and higher |
Optional |
type
Field
type
field means the dialog form of the push message. This form defines how to display push messages on the screen.
The dialogue form of push message has three options.
- Bar type: Bar is displayed on the top of the screen with
icon
,title
, andmessage
. Notification is not displayed when a device screen is off. - Popup Type: Popup is displayed like toast format
- Both Bar and Popup Type: Both bar and popup are displayed on the screen
If a device screen is off and the type is set to Both Bar and Popup Type, popup is displayed only as image above.
bigmsg
and bigpicture
Field
See the image below to check big message and big picture displayed on the screen.
- Use
bigmsg
field to display a bunch of text.ticker
field is available withbigmsg
. - With
bigpicture
field, you can display a big-size picture on the notification screen. bigpicture
is unavailable withbigmsg
.bigpicture
is available withticker
.
buckettype
Field
If several notifications are shown up and the value of buckettype
field is 1 or 2, the stacked notifications are displayed on a section.
bucketsize
means the number of messages exposed on the notification bar.- If the value of
buckettype
is 1, which means Inboxing type, and If the message exceeds one line, an ellipsis (…) is added, and the contents beyond the screen are not displayed. - If the value of
buckettype
is 2, the whole text is displayed on the screen. buckettype
is available withticker
.
Send Facebook Cloud Game Notification (Android)
With the Hive SDK‘s local push feature provided from the Facebook Cloud Game build, you can use Facebook App To User Notifications.
For the Facebook notification, there are limitations which are different from the ordinary pushes of Android. Please check the details on the Facebook App To User Notifications page.
Note that the Facebook notifications features are constantly being updated by Facebook, causing constant changes in its behavior. Until the Facebook notification feature becomes stable, the Hive SDK will be continuously updated.
(Hive v4.16.1 has followed up and verified the Facebook App To User Notifications update as of October 2022.) The data required for the the Facebook notification feature must be defined in the LocalPush class. The following table defines which of the fields of the LocalPush class are required for the Facebook notifications.
NAME | TYPE | DESCRIPTION | REQUIRED |
title | String | – Local push message title– 1~30 characters are required– The title value is required as of Hive 4.16.1, but it is not confirmed where it is used in the Facebook UI. | required |
msg | String | – Local push message content– 10~180 characters are required– Exposed as a message in the notification | required |
after | Integer | – Indicates how many seconds it will take after push registrationto display a push message (in seconds, default value = 0)– Set the time value (seconds) up to 10 days or less | required |
bigpicture | String | – Image setting required– 300×200 px– less than 10MB– If the image is posted as a web link, the url starting with http or https(ex: https://hive-fn.qpyou.cn/hubweb/hive_img/U/P/122349090/20151028/82f610b6f4590863934cefb2b875c87a.jpg)– If the image file is included in project resources, the image file should be included under /res/drawable. If the file name is fbcloudtest.png, only fbcloudtest is entered as a value. | required |
When a notification arrives, the content of the notification is displayed on the Facebook web page and app as shown below.
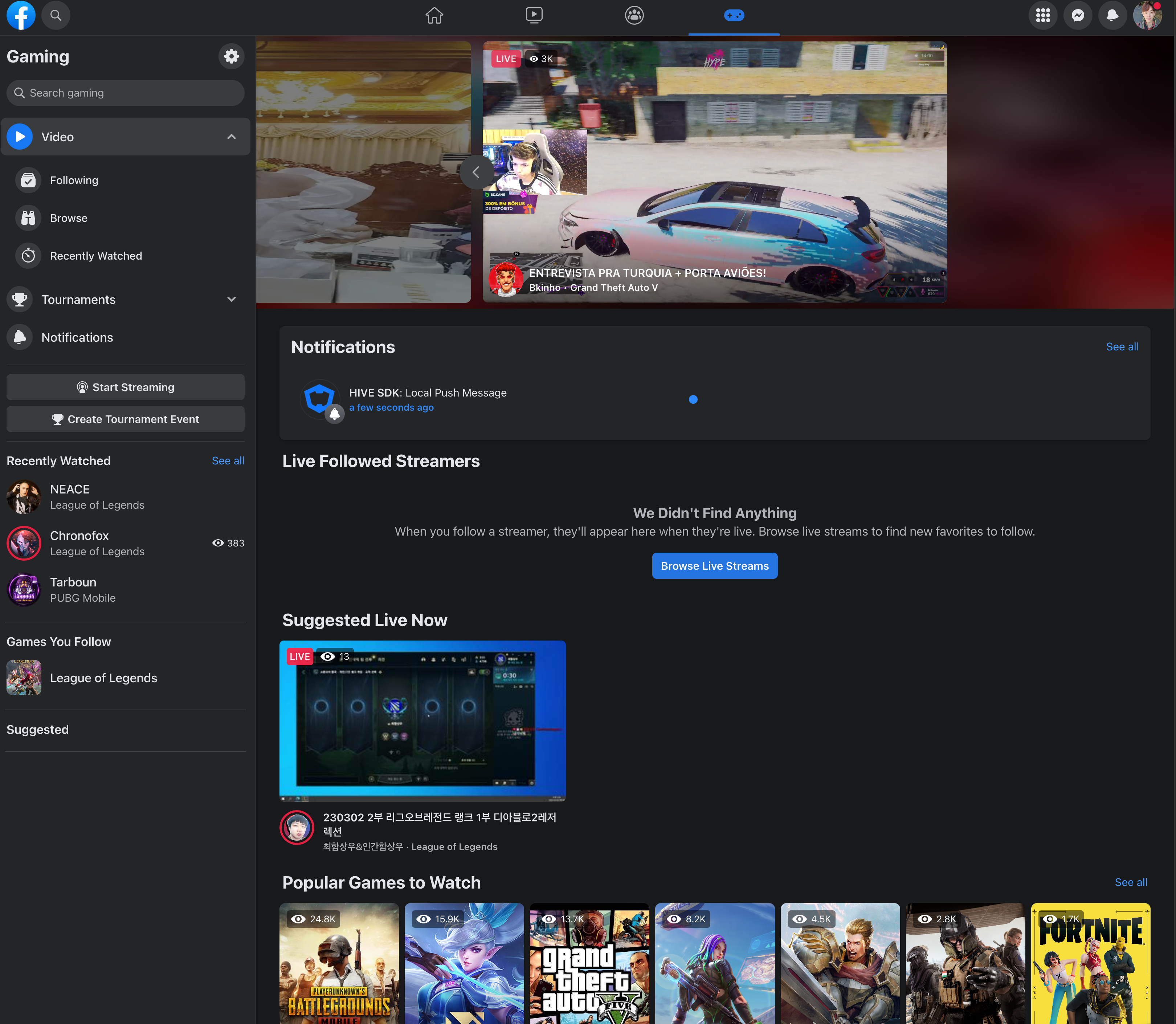
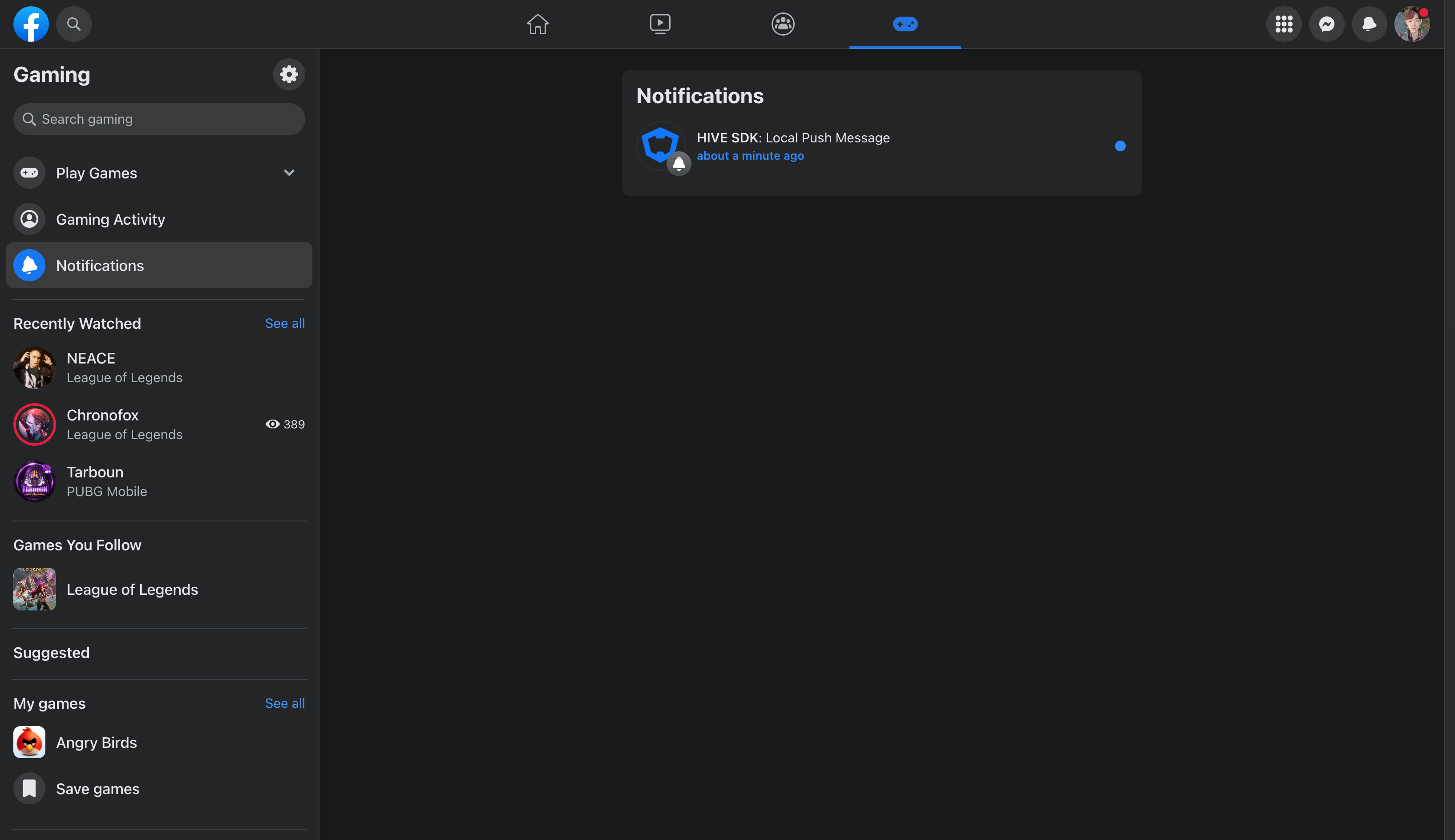
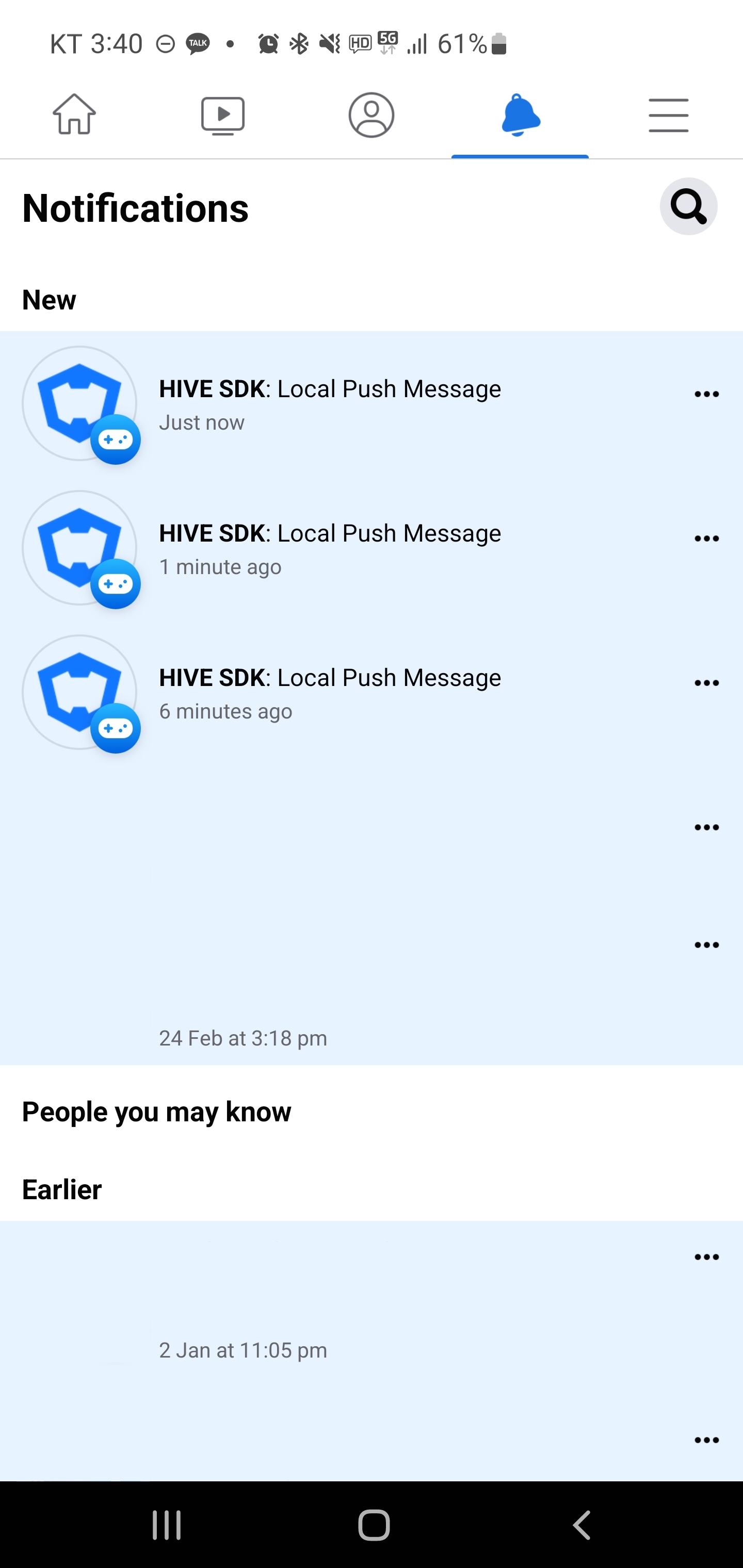