On News page, you can see the event banners and notice of the games registered in Hive console together on one page.
The Coupon Exchange appears on the News page on Android device only.
Configuring News Page
Register the contents of Notice, Cross-promotion and Event banner, which compose News page, on Hive Console. You can specify the sequence of components, for example, whether to place notice at the top or event banners at the top and whether to display the badage notifying the imminent end of an event and the badge notifying the available period one can receive gifts from the Hive console. For more information about registration, see Hive Console Promotion.
Displaying News Page
To show up the news page, set promotionType
as PromotionType.NEWS
and call showPromotion()
method in the Promotion class.
Followings are sample codes to display a news page.
API Reference: hive.Promotion.showPromotion
1 2 3 4 5 6 7 8 9 10 11 12 |
using hive; PromotionType promotionViewType = PromotionType.NEWS; // If true, the 'Do not watch again today' button will not be displayed. Even if the user has already set it not to be viewed again today, it is ignored and the news page is displayed. Boolean isForced = false; Promotion.showPromotion(promotionViewType, isForced, (ResultAPI result, PromotionEventType viewEventType) => { if (result.isSuccess()) { // call successful } }); |
API Reference: Promotion::showPromotion
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
#include <HIVE_SDK_Plugin/HIVE_CPP.h> using namespace std; using namespace hive; PromotionType promotionViewType = PromotionType::NEWS; // If true, the 'Do not watch again today' button will not be displayed. Even if the user has already set it not to be viewed again today, it is ignored and the news page is displayed. bool isForced = false; Promotion::showPromotion(promotionViewType, isForced, [=](ResultAPI result, PromotionEventType viewEventType) { if (result.isSuccess()) { // call successful } }); |
API Reference: Promotion.showPromotion
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
import com.hive.Promotion import com.hive.ResultAPI val promotionViewType = Promotion.PromotionViewType.NEWS // If true, the 'Do not watch again today' button will not be displayed. Even if the user has already set it not to be viewed again today, it is ignored and the news page is displayed. val isForced = false Promotion.showPromotion(promotionViewType, isForced, object : Promotion.PromotionViewListener { override fun onPromotionView(result: ResultAPI, promotionEventType: Promotion.PromotionViewResultType) { if (result.isSuccess) { // call successful } } }) |
API Reference: com.hive.Promotion.showPromotion
1 2 3 4 5 6 7 8 9 10 11 12 13 |
import com.hive.Promotion; import com.hive.ResultAPI; Promotion.PromotionViewType promotionViewType = Promotion.PromotionViewType.NEWS; // If true, the 'Do not watch again today' button will not be displayed. Even if the user has already set it not to be viewed again today, it is ignored and the news page is displayed. boolean isForced = false; Promotion.INSTANCE.showPromotion(promotionViewType, isForced, (result, promotionEventType) -> { if (result.isSuccess()) { // call successful } }); |
API Reference: PromotionInterface.showPromotion
1 2 3 4 5 6 7 8 9 10 11 12 |
import HIVEService let promotionViewType: PromotionViewType = .news // If true, the 'Do not watch again today' button will not be displayed. Even if the user has already set it not to be viewed again today, it is ignored and the news page is displayed. let isForced = false PromotionInterface.showPromotion(promotionViewType, isForced: isForced) { result, viewResultType in if result.isSuccess() { // call successful } } |
API Reference: HIVEPromotion::showPromotion
1 2 3 4 5 6 7 8 9 10 11 12 |
#import <HIVEService/HIVEService-Swift.h> HIVEPromotionViewType promotionViewType = HIVEPromotionViewTypeNews; // If true, the 'Do not watch again today' button will not be displayed. Even if the user has already set it not to be viewed again today, it is ignored and the news page is displayed. BOOL isForced = NO; [HIVEPromotion showPromotion: promotionViewType isForced: isForced handler: ^(HIVEResultAPI *result, HIVEPromotionViewResultType viewResultType) { if ([result isSuccess]) { // call successful } }]; |
- “Do not display News page again” option
You can let users choose not to watch the news page for a day. To activate a checkbox not to show up the page, setisForced
parameter asfalse
when callingshowPromotion()
method in the Promotion class. -
Displaying News page by force
If you do not provide users with the option “Do not display this page again”, or even if the user has already opted not to view it for a day, but if you want to ignore the user’s preferences and display the news page to the user, you can setisForced
parameter totrue
when callingshowPromotion()
method in the Promotion class.If you setisForced
parameter astrue
, News page does not show a checkbox to tick “Do not display this page again”. Even the user already ticked “Do not display this page again”, News page is forcibly shown up.
Displaying News Page with Notice activated
To display the News page with Notice only, set promotionType
as PromotionType.NOTICE
, and call showPromotion()
method in the Promotion class.
Followings are sample codes to display a list of notice.
API Reference: hive.Promotion.showPromotion
1 2 3 4 5 6 7 8 9 10 11 12 |
using hive; PromotionType promotionViewType = PromotionType.NOTICE; // If true, the 'Do not watch again today' button will not be displayed. Even if the user has already set it to not be viewed again today, it will be ignored and the notice page will be displayed. Boolean isForced = false; Promotion.showPromotion(promotionViewType, isForced, (ResultAPI result, PromotionEventType viewEventType) => { if (result.isSuccess()) { // call successful } }); |
API Reference: Promotion ::showPromotion
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
#include <HIVE_SDK_Plugin/HIVE_CPP.h> using namespace std; using namespace hive; PromotionType promotionViewType = PromotionType::NOTICE; // If true, the 'Do not watch again today' button will not be displayed. Even if the user has already set it to not be viewed again today, it will be ignored and the notice page will be displayed. bool isForced = false; Promotion::showPromotion(promotionViewType, isForced, [=](ResultAPI result, PromotionEventType viewEventType) { if (result.isSuccess()) { // call successful } }); |
API Reference: Promotion.showPromotion
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
import com.hive.Promotion import com.hive.ResultAPI val promotionViewType = Promotion.PromotionViewType.NOTICE // If true, the 'Do not watch again today' button will not be displayed. Even if the user has already set it to not be viewed again today, it will be ignored and the notice page will be displayed. val isForced = false Promotion.showPromotion(promotionViewType, isForced, object : Promotion.PromotionViewListener { override fun onPromotionView(result: ResultAPI, promotionEventType: Promotion.PromotionViewResultType) { if (result.isSuccess) { // call successful } } }) |
API Reference: Promotion .INSTANCE.showPromotion
1 2 3 4 5 6 7 8 9 10 11 12 13 |
import com.hive.Promotion; import com.hive.ResultAPI; Promotion.PromotionViewType promotionViewType = Promotion.PromotionViewType.NOTICE; // If true, the 'Do not watch again today' button will not be displayed. Even if the user has already set it to not be viewed again today, it will be ignored and the notice page will be displayed. boolean isForced = false; Promotion.INSTANCE.showPromotion(promotionViewType, isForced, (result, promotionEventType) -> { if (result.isSuccess()) { // call successful } }); |
API Reference: PromotionInterface.showPromotion
1 2 3 4 5 6 7 8 9 10 11 12 |
import HIVEService let promotionViewType: PromotionViewType = .notice // If true, the 'Do not watch again today' button will not be displayed. Even if the user has already set it to not be viewed again today, it will be ignored and the notice page will be displayed. let isForced = false PromotionInterface.showPromotion(promotionViewType, isForced: isForced) { result, viewResultType in if result.isSuccess() { // call successful } } |
API Reference: HIVEPromotion showPromotion
1 2 3 4 5 6 7 8 9 10 11 12 |
#import <HIVEService/HIVEService-Swift.h> HIVEPromotionViewType promotionViewType = HIVEPromotionViewTypeNotice; // If true, the 'Do not watch again today' button will not be displayed. Even if the user has already set it to not be viewed again today, it will be ignored and the notice page will be displayed. BOOL isForced = NO; [HIVEPromotion showPromotion: promotionViewType isForced: isForced handler: ^(HIVEResultAPI *result, HIVEPromotionViewResultType viewResultType) { if ([result isSuccess]) { // call successful } }]; |
Displaying News Page with a particular menu activated
To display the News page with a particular menu, call showNews()
method in the Promotion class with promotionType
as a parameter which registered in setting News page.
Followings are sample codes to display the News page with a particular menu activated.
API Reference: hive .Promotion.showNews
1 2 3 4 5 6 7 8 9 |
using hive; String menu = "Promotion type registered in Hive console"; Promotion.showNews(menu, (ResultAPI result, PromotionEventType viewEventType) => { if (result.isSuccess()) { // call successful } }); |
API Reference: Promotion ::showNews
1 2 3 4 5 6 7 8 9 10 11 |
#include <HIVE_SDK_Plugin/HIVE_CPP.h> using namespace std; using namespace hive; string menu = "Promotion type registered in Hive console"; Promotion::showNews(menu, [=](ResultAPI result, PromotionEventType viewEventType) { if (result.isSuccess()) { // call successful } }); |
API Reference: Promotion.showNews
1 2 3 4 5 6 7 8 9 10 11 12 |
import com.hive.Promotion import com.hive.ResultAPI val menu = "Promotion type registered in Hive console" Promotion.showNews(menu, null, object : Promotion.PromotionViewListener { override fun onPromotionView(result: ResultAPI, promotionEventType: Promotion.PromotionViewResultType) { if (result.isSuccess) { // call successful } } }) |
API Reference: Promotion .INSTANCE.showNews
1 2 3 4 5 6 7 8 9 10 |
import com.hive.Promotion; import com.hive.ResultAPI; String menu = "Promotion type registered in Hive console"; Promotion.INSTANCE.showNews(menu, null, (result, promotionEventType) -> { if (result.isSuccess()) { // call successful } }); |
API Reference: PromotionInterface.showNews
1 2 3 4 5 6 7 8 9 |
import HIVEService let menu = "Promotion type registered in Hive console"; PromotionInterface.showNews(menu) { result, viewResultType in if result.isSuccess() { // call successful } } |
API Reference: HIVEPromotion showNews
1 2 3 4 5 6 7 8 9 |
#import <HIVEService/HIVEService-swift.h> NSString *menu = @"Promotion type registered in Hive console"; [HIVEPromotion showNews: menu handler: ^(HIVEResultAPI *result, HIVEPromotionViewResultType viewResultType) { if ([result isSuccess]) { // call successful } }]; |
Display a News Page Highlighting an Achieved Event Banner
To display the news page highlighting accomplished events, use the achieved event banner number (pid
) as a parameter when calling the Promotion class showNews()
method. The following is an example code to display a news page highlighting the achieved event banner when a gamer achieved a specific event.
API Reference: hive.Promotion.showNews
1 2 3 4 5 6 7 8 9 |
// Set the parameter to activate the event menu String menu = "event"; // Set the Pid list for the achieved event banner to distinguish the achieved event banner. List<int> giftPidList = [101331, 121881, 253120, 100002]; // Pid example // the callback handler of the result of exposing the news page with the event menu activated public void onPromotionViewCB(ResultAPI result, PromotionEventType promotionEventType) { if(result.isSuccess()){ // API call succeeded } } // Expose the news page with the event menu activated hive.Promotion.showNewsmenu, giftPidList, onPromotionViewCB); |
API Reference: Promotion::showCustomContents
1 2 3 4 5 6 7 8 9 10 |
// Set the parameter to activate the event menu string menu = "event"; // Set the Pid list for the achieved event banner to distinguish the achieved event banner. std::vector<int> giftPidList = {101331, 121881, 253120, 100002}; // Pid example // Expose the news page with the event menu activated Promotion::showNewsmenu, giftPidList, [=]ResultAPI result, PromotionEventType promotionEventType){ // the callback handler of the result of exposing the news page with the event menu activated if(result.isSuccess()){ // API call succeeded } }); |
API Reference: com.hive.Promotion.showCustomContents
1 2 3 4 5 6 7 8 9 10 11 12 13 |
// Set the parameter to activate the event menu val menu: String = "event" // Set the Pid list for the achieved event banner to distinguish the achieved event banner. val giftPidList = arrayListOf(101331, 121881, 253120, 100002) // Pid Example // Expose the news page with the event menu activated Promotion.showNewsmenu, giftPidList, object : Promotion.PromotionViewListener { override fun onPromotionViewresult: ResultAPI, promotionEventType: Promotion.PromotionViewResultType) { // the callback listener of the result of exposing the news page with the event menu activated if (result.isSuccess) { // Successful API Call } } } |
API Reference: com.hive.Promotion.showCustomContents
1 2 3 4 5 6 7 8 |
// Set the parameter to activate the event menu String menu = "event"; // Set the pid list for the achieved event banner to distinguish the achieved event banner. ArrayList<Integer> giftPidList = new ArrayList<Integer>(Arrays.asList1, 2, 3, 4)); // Expose the news page with the event menu activated Promotion.showNewsmenu, giftPidList, new PromotionViewListener) { @Override public void onPromotionView@NotNull ResultAPI result, @NotNull PromotionViewResultType promotionEventType) { // the callback listener of the result of exposing the news page with the event menu activated if (result.isSuccess) { // API call succeeded } } }); |
API Reference: HivePromotion:showCustomContents
1 2 3 4 5 6 7 8 9 10 11 |
// Set the parameter to activate the event menu NSString *menu = @"event"; // Set the Pid list for the achieved event banner to distinguish the achieved event banner. NSArray *giftPidList = @[@101331, @121881, @253120, @100002]; // Pid example // Expose the news page with the event menu activated [HIVEPromotion showNewsWithMenu:menu giftPidList:giftPidList handler:^HIVEResultAPI * result, HIVEPromotionViewResultType type) { // the callback listener of the result of exposing the news page with the event menu activated if (result.isSuccess) { // Successful API Call } }]; |
API Reference: HivePromotion:showCustomContents
1 2 3 4 5 6 7 8 9 10 11 |
// Set the parameter to activate the event menu String menu = "event" // Set the Pid list for the achieved event banner to distinguish the achieved event banner. let giftPidList = [101331, 121881, 253120, 100002] // Example Pid // Expose the news page with the event menu activated HivePromotion.showNewsmenu: menu, giftPidList: giftPidList) { result, type in // the callback listener of the result of exposing the news page with the event menu activated if result.isSuccess { // Successful API Call } } |
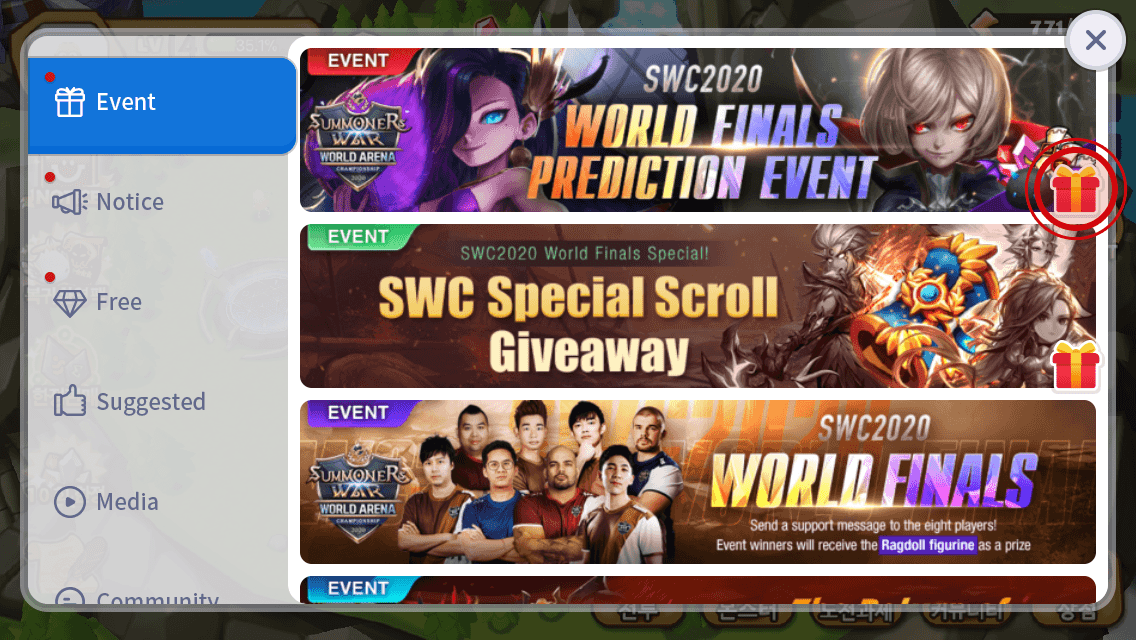
Adding Data Renewal API
News page data is sent through a communication with Hive server after sign-in. If user changes the settings in game such as game language and game server, data renewal is needed. Data may not be updated to the latest if user goes on a long gaming binge. In these cases, game studio is required to manually renew the data on News page when user access to game lobby. Call updatePromotionData()
method in the Promotion class for data renewal.
Followings are sample codes to renew the News data.
API Reference: hive.Promotion.updatePromotionData
1 2 3 |
using hive; Promotion.updatePromotionData(); |
API Reference: Promotion::updatePromotionData
1 2 3 4 5 |
#include <HIVE_SDK_Plugin/HIVE_CPP.h> using namespace std; using namespace hive; Promotion::updatePromotionData(); |
API Reference: Promotion.updatePromotionData
1 2 3 |
import com.hive.Promotion Promotion.updatePromotionData() |
API Reference: Promotion.INSTANCE.updatePromotionData
1 2 3 |
import com.hive.Promotion; Promotion.INSTANCE.updatePromotionData(); |
API Reference: PromotionInterface.updatePromotionData
1 2 3 |
import HIVEService PromotionInterface.updatePromotionData() |
API Reference: HIVEPromoiton updatePromotionData
1 2 3 |
#import <HIVEService/HIVEService-Swift.h> [HIVEPromoiton updatePromotionData]; |
Adding improved Data Renewal API
- The logic of calling 'updatePromotionData API' after calling 'setServerID API' has been improved so that the same operation is performed by calling the updateServerID API only once.
- The logic of calling 'pdatePromotionData API' after calling 'setGameLanguage API' has been improved so that it works the same by calling the 'updateGameLanguage API' only once.
The following is an example code to add the improved Data Renewal API
API Reference: hive.Promotion.updateServerId
API Reference: hive.Promotion.updateGameLanguage
1 2 3 |
using hive; Configuration.updateServerId("server_001"); Configuration.updateGameLanguage("en"); |
API Reference: Promotion.updateServerId
API Reference: Promotion.updateGameLanguage
1 2 3 4 5 6 |
#include <HIVE_SDK_Plugin/HIVE_CPP.h> using namespace std; using namespace hive; Configuration::updateServerId("server_001"); Configuration::updateGameLanguage("en"); |
API Reference: Configuration.updateServerId
API Reference: Configuration.updateGameLanguage
1 2 3 4 |
import com.hive.Configuration Configuration.updateServerId("server_001") Configuration.updateGameLanguage("en") |
API Reference: com.hive.Promotion.updateServerId
API Reference: com.hive.Promotion.updateGameLanguage
1 2 3 4 |
import com.hive.Configuration; Configuration.INSTANCE.updateServerId("server_001"); Configuration.INSTANCE.updateGameLanguage("en"); |
API Reference: ConfigurationInterface .updateServerId
API Reference: ConfigurationInterface .updateGameLanguage
1 2 3 4 |
import HIVEService ConfigurationInterface.updateServerId("server_001") ConfigurationInterface.updateGameLanguage("en") |
API Reference: HIVEPromotion::updateServerId
API Reference: HIVEPromotion::updateGameLanguage
1 2 3 4 |
#import <HIVEService/HIVEService-Swift.h> [HIVEConfiguration updateServerId: @"server_001"]; [HIVEConfiguration updateGameLanguage: @"en"]; |